Project Management¶
Project Quick Start¶
Project and Project Manager Quick Start¶
You can work directly on project:
project1 = Project(project_name="mynewproj", project_path="/path/to/proj")
project1.rename("project", "mynewproj", "hello_project")
project1.import()
project1.authors = "OpenAlea Consortium and John Doe"
project1.description = "Test project concept with numpy"
project1.long_description = '''This project import numpy.
Then, it create and display a numpy eye.
We use it to test concept of Project.''
project1.add(category="scripts", name"hello.py", value="print 'Hello World'")
project1.description = "This project is used to said hello to everyone"
project1.add("startup", "begin_numpy.py", "import numpy as np")
project1.add("scripts", "eye.py", "print np.eye(2)")
project1.rename("scripts", "eye.py", "eye_numpy.py")
project1.save()
Or, you can create or load a project thanks to the project manager.
from openalea.vpltk.project.manager import ProjectManager
# Instanciate ProjectManager
project_manager = ProjectManager()
# Discover available projects
project_manager.discover()
print project_manager.projects
# Create project in default directory or in specific one
p1 = project_manager.create('project1')
p2 = project_manager.create('project2', '/path/to/project')
# Load project from default directory or in specific one
p3 = project_manager.load('project3')
p4 = project_manager.load('project4', '/path/to/project')
# Load
project2 = project_manager.load("numpy_project")
# Run startup
project2.import()
# Run script
project2.run_script("eye_numpy.py")
To search projects that are not located inside default directories:
project_manager.find_links.append('path/to/search/projects')
project_manager.discover()
print project_manager.projects
Various Views on Project Manager and Project¶
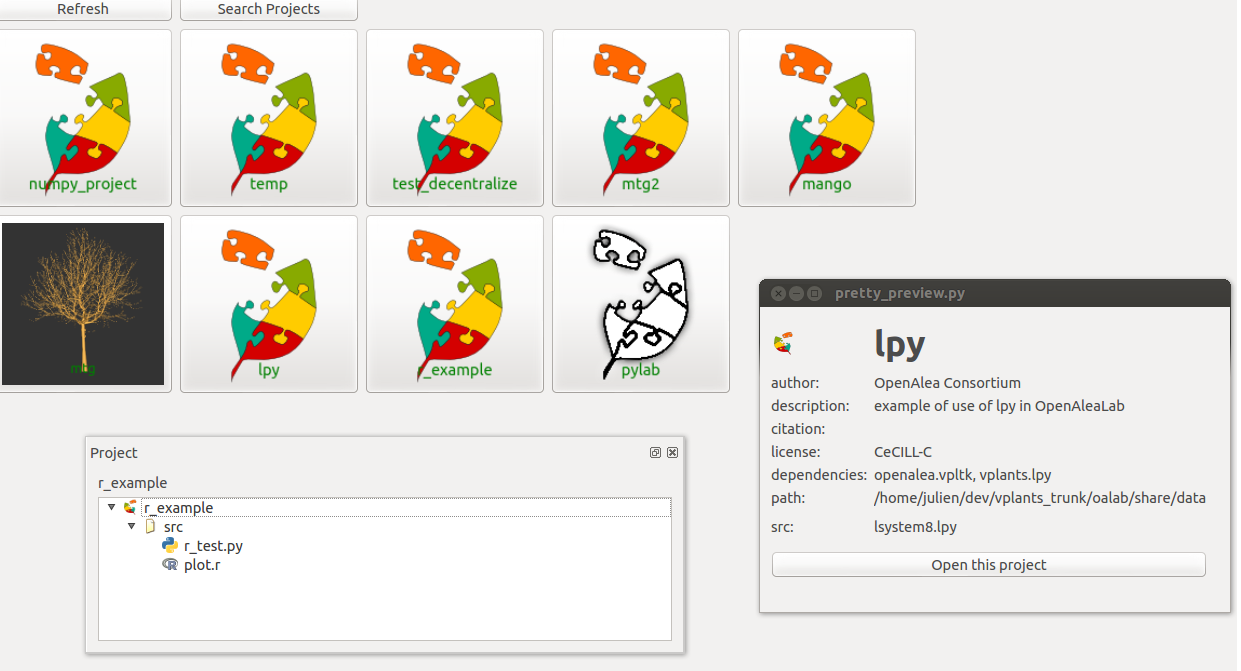
Project API¶
The Project is a structure which permit to manage different objects.
It store metadata (name, author, description, version, license, ...) and data (src, models, images, ...).
You have here the default architecture of the project named “project_name”, stored in your computer.
/project_name | oaproject.cfg (Configuration file) /src (Files sources, Script Python, LPy...) /control (Control, like color map or curve) /scene (scene, scene 3D) /cache (Intermediary saved objects) /data (Data files like images, .dat, ...) /startup (Preprocessing scripts)
|
use: | project1 = Project(project_name="mynewproj", project_path="/path/to/proj")
project1.start()
project1.add(category="src", name"hello.py", value="print 'Hello World'")
project1.author = "John Doe"
project1.description = "This project is used to said hello to everyone"
project1.save()
|
---|
- class openalea.vpltk.project.project.Project(project_name, project_path, icon='', author='OpenAlea Consortium', author_email='', description='', long_description='', citation='', url='', dependencies=, []license='CeCILL-C', version='0.1')[source]¶
- add(category, name, value)[source]¶
Add an object in the project
Parameters: - category – type of object to add (“src”, “control”, “scene”, ...)
- name – filename of the object to add (path or str)
- value – to add (string)
- add_script(name, script)[source]¶
Add a src in the project
Deprecated: replace by add() method
Parameters: - name – filename of the src to add (path or str)
- script – to add (string)
See also
- get(category, name)[source]¶
Search an object inside project and return it.
Parameters: - category – category of object to get
- name – name of object to get
Returns: object named name in the category category if it exists. Else, None.
Use: >>> get(category="src", name="myscript.py")
See also
- load()[source]¶
Realize a total loading of project (contrary to load_manifest()).
- Load manifest load_manifest()
- Read data files listed in manifest and fill project object.
See also
- load_manifest()[source]¶
Partially load a project from a manifest file.
- Read manifest file (oaproject.cfg).
- Load metadata inside project from manifest.
- Load filenames of data files inside project from manifest.
- Not load data ! If you want to load data, please use load().
Warning: load metadata and list of filenames but does not load files See also
- remove(category, name)[source]¶
Remove an object in the project
Remove nothing on disk.
Parameters: - category – category of object to remove (“src”, “control”, “scene”, ...) (str)
- name – filename of the src to remove (path or str)
- remove_script(name)[source]¶
Add a src in the project
Remove nothing on disk.
Deprecated: replace by remove() method Parameters: name – filename of the src to remove (path or str) See also
- rename(category, old_name, new_name)[source]¶
Rename a src, a scene or a control in the project. If category is project, rename the entire project.
Parameters: - category – Can be “src”, “control”, “scene” or “project” (str)
- old_name – current name of thing to rename (str)
- new_name – future name of thing to rename (str)
- save()[source]¶
Save project on disk.
- Save data files.
- Save metadata and list on previously saved data into a manifest file (oaproject.cfg).
See also
- save_manifest()[source]¶
Save a manifest file on disk. His name is “oaproject.cfg”.
It contains list of files that are inside project (manifest) and metadata (author, version, ...).
See also
- class openalea.vpltk.project.manager.ProjectManager[source]¶
Object which manage projects: creation, loading, saving, searching, ...
It is a singleton.
- create(project_name, project_path=None)[source]¶
Create new project and return it.
Use: >>> project1 = project_manager.create('project1') >>> project2 = project_manager.create('project2', '/path/to/project')
Parameters: - project_name – name of project to create (str)
- project_path – path where project will be saved. By default, project_path is the user path of all projects ($HOME/.openalea/projects/).
Returns: Project
- discover()[source]¶
Discover projects from your disk and put them in self.projects.
Projects are not loaded, only metadata are.
Use: >>> project_manager.discover() >>> print project_manager.projects
To discover new projects, you can add path into self.find_links
project_manager.find_links.append('path/to/search/projects') project_manager.discover()
- get_current()[source]¶
Returns: current active project
Use: >>> project = project_manager.get_current()
- instance = None¶
- load(project_name, project_path=None)[source]¶
Load existing project
Use: >>> project1 = project_manager.load('project1') >>> project2 = project_manager.load('project2', '/path/to/project')
Parameters: - project_name – name of project to load. Must be a string.
- project_path – path of project to load. Must be a path (see module path.py). By default, the path is the openaelea.core.settings.get_project_dir() ($HOME/.openalea/projects/).
Returns: Project